Parallax Hero
An interactive hero section with dynamic effects and mouse-driven animations.
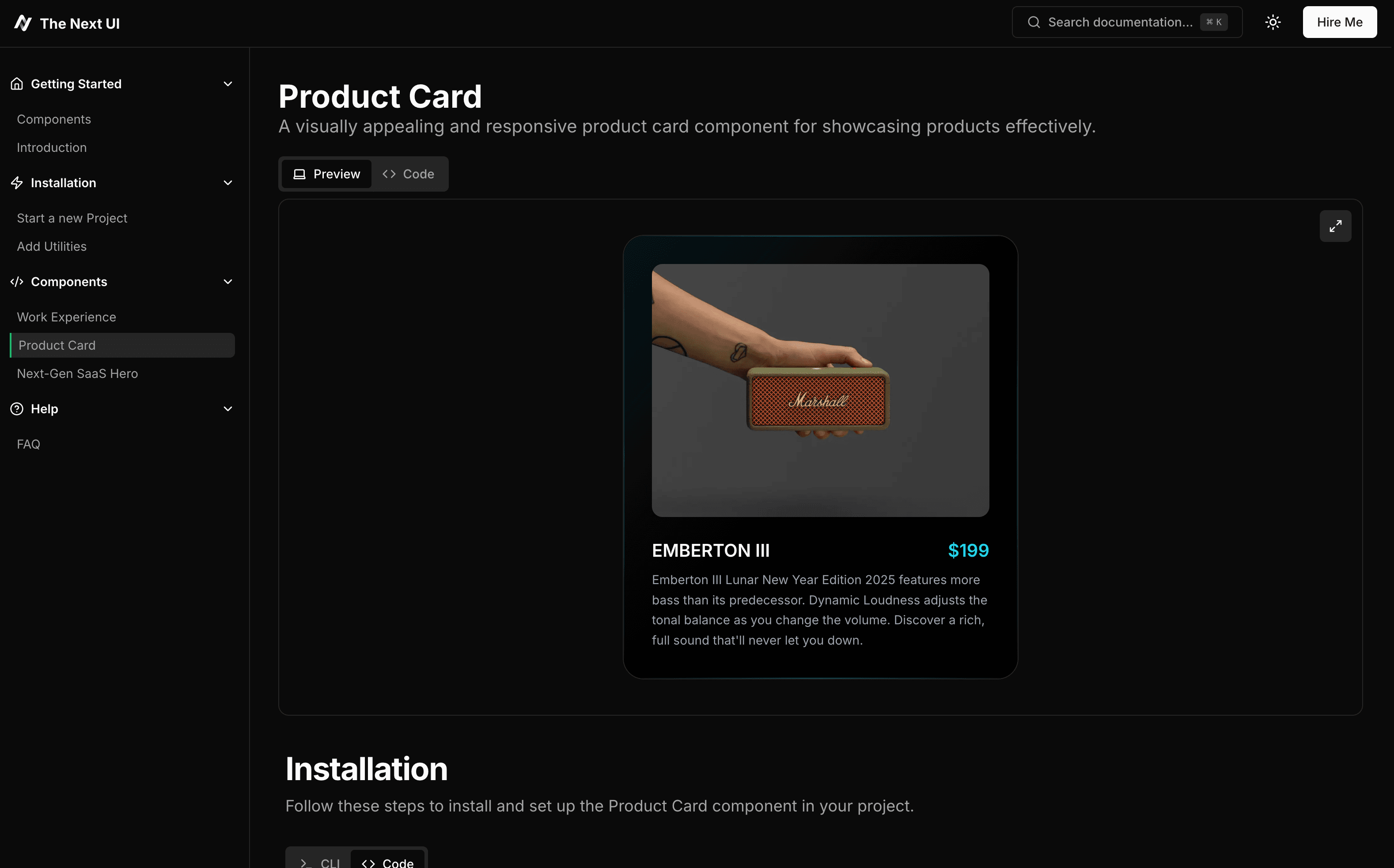
Installation
Follow these steps to install and set up the Hero component in your project.
1
Install dependencies
Install the required dependencies like motion
to use this component.
npm install motion
2
Add the Hero component to your page
Finally, create the component file.
/components/Parallaxhero.tsx
"use client"
import { motion, useScroll, useTransform } from "motion/react"
import { useState, useEffect, useRef } from "react"
import Image from "next/image"
import Link from "next/link"
interface HeroProps {
title?: string
description?: string
previewImage?: string
buttonText?: string
slug?: string
}
export default function ParallaxHero({
title = "Meet The Next UI",
description = "Premium, editable components for modern web development.",
previewImage = "/CompsPreview/taskk-dashboard.png",
buttonText = "Try Demo",
slug = '/components/parallax-hero'
}: HeroProps) {
const [mousePosition, setMousePosition] = useState({ x: 0, y: 0 })
const previewRef = useRef(null)
const [isHovered, setIsHovered] = useState(false);
const { scrollYProgress } = useScroll({
target: previewRef,
offset: ["start end", "end start"]
})
const y = useTransform(scrollYProgress, [0, 1], ["0%", "20%"])
const scale = useTransform(scrollYProgress, [0, 0.5, 1], [1, 1.05, 1.1])
useEffect(() => {
const handleMouseMove = (e: MouseEvent) => {
setMousePosition({
x: (e.clientX / window.innerWidth) * 2 - 1,
y: (e.clientY / window.innerHeight) * 2 - 1,
})
}
window.addEventListener("mousemove", handleMouseMove)
return () => window.removeEventListener("mousemove", handleMouseMove)
}, [])
return (
<section className="relative min-h-screen flex flex-col items-center justify-center px-4 py-20 bg-[#0A0415]/10 overflow-hidden">
<div className="absolute inset-0">
<div
className="absolute inset-0 opacity-[0.40]"
style={{
backgroundImage: `
linear-gradient(90deg, rgba(123, 97, 255, 0.1) 1px, transparent 1px),
linear-gradient(rgba(123, 97, 255, 0.1) 1px, transparent 1px)
`,
backgroundSize: "30px 30px",
transform: "perspective(1000px) rotateX(60deg) scale(2.5) translateY(-10%)",
transformOrigin: "center center",
}}
/>
<div
className="absolute inset-0"
style={{
background: `radial-gradient(circle at ${50 + mousePosition.x * 10}% ${50 + mousePosition.y * 10}%, rgba(123, 97, 255, 0.15) 0%, rgba(123, 97, 255, 0) 50%)`,
filter: "blur(40px)",
transition: "all 0.3s ease-out",
}}
/>
</div>
<div className="absolute inset-0">
<div className="absolute top-0 left-1/4 w-1/2 h-1/2 bg-gradient-to-b from-[rgba(123,97,255,0.1)] to-transparent opacity-30 blur-3xl" />
<div className="absolute bottom-0 left-1/4 w-1/2 h-1/2 bg-gradient-to-t from-[rgba(123,97,255,0.1)] to-transparent opacity-30 blur-3xl" />
</div>
{/* Main Content */}
<div className="container relative z-10 mx-auto max-w-6xl px-4 mt-5">
<motion.div
initial={{ opacity: 0, y: 20 }}
animate={{ opacity: 1, y: 0 }}
transition={{ duration: 0.8, ease: [0.16, 1, 0.3, 1] }}
className="text-center space-y-6"
>
<motion.h1
className="text-4xl md:text-6xl lg:text-7xl font-bold tracking-[-0.02em] text-white"
style={{
textShadow: "0 0 40px rgba(123, 97, 255, 0.2)",
}}
initial={{ opacity: 0, y: 20 }}
animate={{ opacity: 1, y: 0 }}
transition={{ duration: 0.8, delay: 0.2, ease: [0.16, 1, 0.3, 1] }}
>
{title}
</motion.h1>
<motion.div
initial={{ opacity: 0, y: 20 }}
animate={{ opacity: 1, y: 0 }}
transition={{ duration: 0.8, delay: 0.4, ease: [0.16, 1, 0.3, 1] }}
className="space-y-4"
>
<p className="text-lg md:text-xl text-gray-300/40">
{description}
</p>
</motion.div>
<motion.div
initial={{ opacity: 0, y: 20 }}
animate={{ opacity: 1, y: 0 }}
transition={{ duration: 0.8, delay: 0.6, ease: [0.16, 1, 0.3, 1] }}
className="max-w-xl mx-auto mt-8"
>
<motion.div
initial={{ opacity: 0, y: 20 }}
animate={{ opacity: 1, y: 0 }}
transition={{ duration: 0.8, delay: 0.6, ease: [0.16, 1, 0.3, 1] }}
className="max-w-xl mx-auto mt-8"
>
<Link
href={slug}
className="group relative inline-block"
>
<motion.div
className={`
h-14 px-10 py-4 rounded-full text-xl font-semibold
bg-gradient-to-r from-[#7B61FF] to-[#9C8FFF]
text-white shadow-lg shadow-purple-500/20
flex items-center justify-center
relative overflow-hidden
transition-all duration-300
w-fit
`}
initial={{ scale: 1 }}
whileHover={{
scale: 1.05,
width: 'auto',
transition: { duration: 0.5 }
}}
onMouseEnter={() => setIsHovered(true)}
onMouseLeave={() => setIsHovered(false)}
>
{/* Hover effect layer */}
<motion.div
className="absolute inset-0 bg-white/20 opacity-0 group-hover:opacity-100 transition-opacity duration-500"
initial={{ opacity: 0 }}
animate={{ opacity: isHovered ? 0.2 : 0 }}
/>
{/* Button content */}
<div className="relative z-10 flex items-center space-x-2">
<span>{buttonText}</span>
{/* Animated SVG Arrow */}
<motion.svg
xmlns="http://www.w3.org/2000/svg"
width="32"
height="32"
viewBox="0 0 24 24"
fill="none"
stroke="currentColor"
strokeWidth="2"
strokeLinecap="round"
strokeLinejoin="round"
initial={{ x: -10, opacity: 0 }}
animate={{
x: isHovered ? 0 : -10,
opacity: isHovered ? 1 : 0
}}
transition={{
type: "spring",
stiffness: 300,
damping: 20
}}
>
<motion.path
d="M5 12h14"
initial={{ pathLength: 0 }}
animate={{ pathLength: isHovered ? 1 : 0 }}
transition={{ duration: 0.5 }}
/>
<motion.path
d="M12 5l7 7-7 7"
initial={{ pathLength: 0 }}
animate={{ pathLength: isHovered ? 1 : 0 }}
transition={{ duration: 0.5, delay: 0.2 }}
/>
</motion.svg>
</div>
</motion.div>
</Link>
</motion.div>
</motion.div>
</motion.div>
<motion.div
ref={previewRef}
style={{ y, scale }}
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
transition={{ duration: 2, delay: 0.8, ease: [0.16, 1, 0.3, 1] }}
className="relative mt-16 group perspective-1000 max-w-5xl mx-auto"
>
<div className="absolute -inset-1 bg-gradient-to-r from-[#7B61FF] to-[#9C8FFF] rounded-xl opacity-30 blur-2xl group-hover:opacity-50 transition-opacity duration-500" />
<div className="absolute -inset-2 bg-gradient-to-r from-[#7B61FF] to-[#9C8FFF] rounded-xl opacity-20 blur-3xl group-hover:opacity-40 transition-opacity duration-500" />
<div className="relative rounded-xl overflow-hidden shadow-2xl border-4 border-[#7B61FF] group-hover:border-[#9C8FFF] transition-colors duration-500">
<Image
height={1000}
width={1600}
src={previewImage}
alt="Dashboard Preview"
className="w-full h-auto rounded-xl transform transition-transform duration-700 group-hover:scale-[1.01] p-1"
/>
<div className="absolute inset-0 bg-gradient-to-t from-[#0A0415] via-transparent to-[#0A0415] opacity-50" />
</div>
</motion.div>
</div>
</section>
)
}
3
Add the component code
Copy and paste the component code into your project. The component is named Hero
and supports dynamic props such as title, description, and previewImage.
import ParallaxHero from "@/components/Parallaxhero"
Props
Name | Type | Default | Description |
---|---|---|---|
title | string | - | Sets the title text for the hero section. |
description | string | - | Sets the description text for the hero section. |
previewImage | string | - | Sets the image URL for the preview in the hero section. |
buttonText | string | - | Sets the text for the action button. |
slug | string | - | Sets link for the action button. |