Preview Box
This is a preview-type component for dashboards and more, featuring smooth animations.
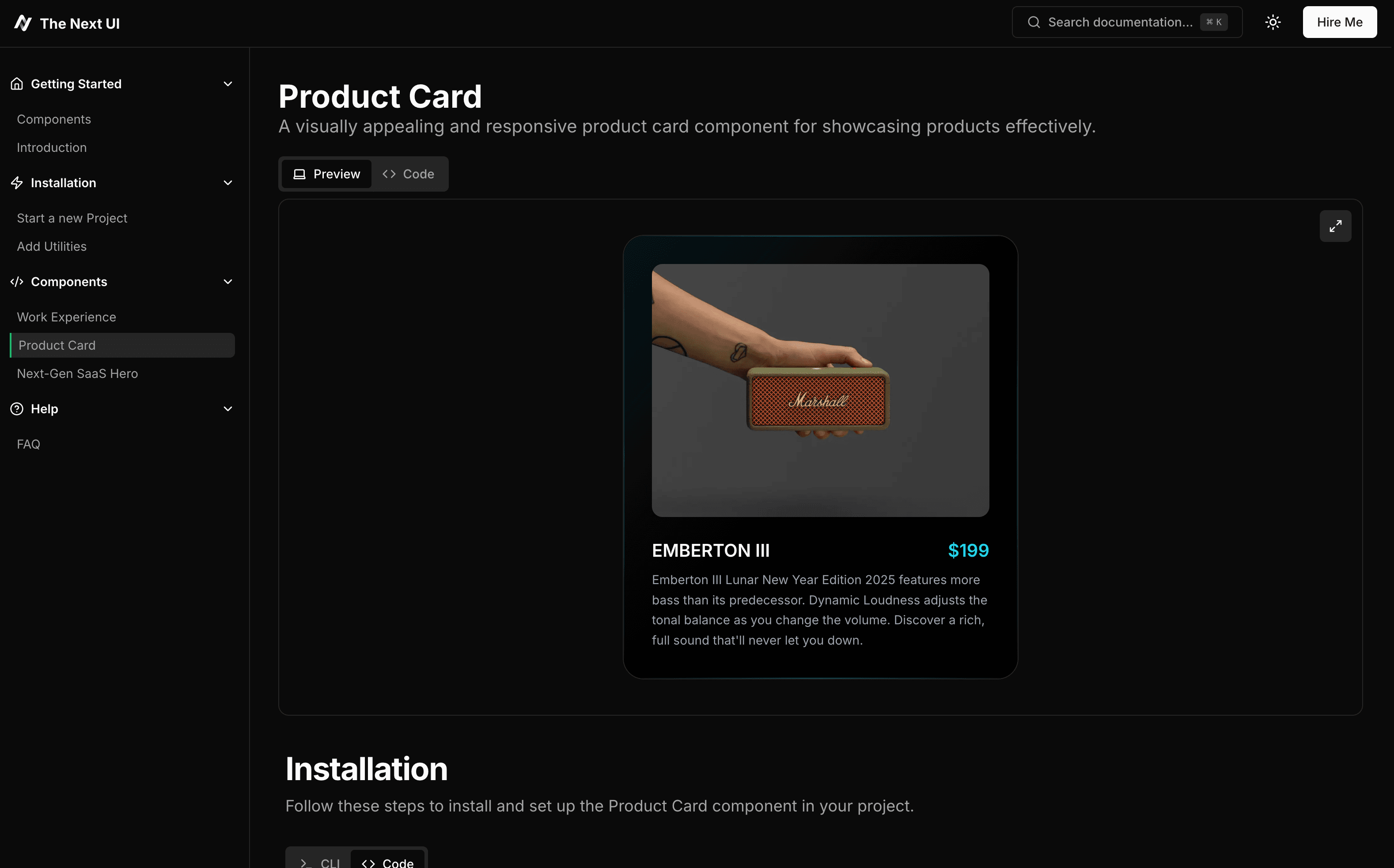
Installation
Follow these steps to install and set up the component in your project.
1
Install dependencies
Install the required dependencies like motion
and next/image
to use this component.
npm install motion
2
Add the component to your page
Finally, create the component file.
components/preview-box.tsx
"use client"
import { useState } from "react"
import Image from "next/image"
import { motion } from "motion/react"
interface PreviewBoxProps {
imagePath: string;
}
export default function PreviewBox({ imagePath }: PreviewBoxProps) {
const [isHovered, setIsHovered] = useState(false)
return (
<div className="sm:min-h-screen h-fit p-4 sm:py-0 py-10 flex items-center justify-center overflow-hidden relative">
{/* Enhanced background with mesh */}
<div className="fixed inset-0 pointer-events-none">
<div className="absolute inset-0 bg-[radial-gradient(circle_at_50%_50%,rgba(17,24,39,1),rgba(0,0,0,1))]" />
<div className="absolute inset-0 bg-[url('data:image/svg+xml;base64,PHN2ZyB3aWR0aD0iMjAiIGhlaWdodD0iMjAiIHhtbG5zPSJodHRwOi8vd3d3LnczLm9yZy8yMDAwL3N2ZyI+PGRlZnM+PHBhdHRlcm4gaWQ9ImdyaWQiIHdpZHRoPSIyMCIgaGVpZ2h0PSIyMCIgcGF0dGVyblVuaXRzPSJ1c2VyU3BhY2VPblVzZSI+PHBhdGggZD0iTSAwIDEwIEwgMjAgMTAgTSAxMCAwIEwgMTAgMjAiIGZpbGw9Im5vbmUiIHN0cm9rZT0icmdiYSgyNTUsMjU1LDI1NSwwLjAzKSIgc3Ryb2tlLXdpZHRoPSIxIi8+PC9wYXR0ZXJuPjwvZGVmcz48cmVjdCB3aWR0aD0iMTAwJSIgaGVpZ2h0PSIxMDAlIiBmaWxsPSJ1cmwoI2dyaWQpIi8+PC9zdmc+')] opacity-40" />
</div>
{/* Main container */}
<motion.div
className="relative max-w-4xl w-full rounded-xl overflow-hidden p-2"
onMouseEnter={() => setIsHovered(true)}
onMouseLeave={() => setIsHovered(false)}
animate={{
scale: isHovered ? 1.02 : 1,
rotate: isHovered ? 1 : 0,
}}
transition={{
type: "spring",
stiffness: 200,
damping: 20,
}}
>
{/* Dynamic glow effects */}
<motion.div
className="absolute inset-0 rounded-xl opacity-80 blur-2xl"
animate={{
background: isHovered
? "radial-gradient(circle at bottom right, rgb(59, 130, 246), transparent 70%), radial-gradient(circle at top left, rgb(249, 115, 22), transparent 70%)"
: "radial-gradient(circle at top left, rgb(59, 130, 246), transparent 70%), radial-gradient(circle at bottom right, rgb(249, 115, 22), transparent 70%)",
rotate: isHovered ? 180 : 0,
}}
transition={{
duration: 0.8,
ease: "easeInOut",
}}
/>
{/* Content */}
<div className="relative rounded-xl overflow-hidden backdrop-blur-sm border border-gray-800/50 bg-gray-900/90">
<Image src={imagePath} alt="Preview" width={800} height={500} layout="responsive" className="h-auto w-full" />
</div>
</motion.div>
</div>
)
}
3
Add the component code
Copy and paste the component code into your project. The component is named PreviewBox
and uses dynamic props.
import { PreviewBox } from "@/components/preview-box
Props
Name | Type | Default | Description |
---|---|---|---|
imagePath* | string | - | The path to the image to be displayed in the preview box. |